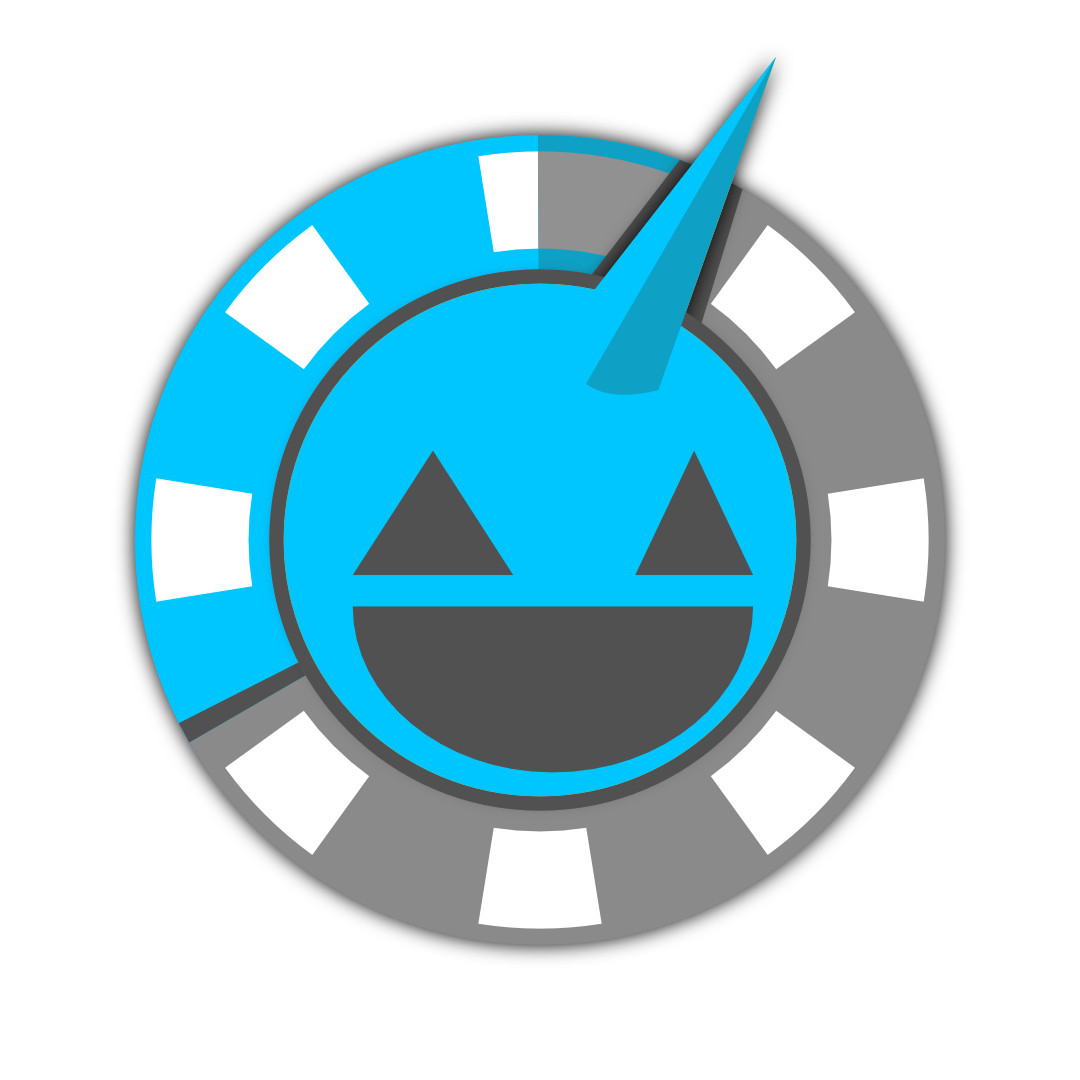 |
PokerUnicorn
|
Loading...
Searching...
No Matches
Go to the documentation of this file.
21#define STRINGIFY(x) #x
22#define TOSTRING(x) STRINGIFY(x)
24#define VARCAT(left, right) left##right
26#define LIST_FOREACH(list, node) { \
27 int VARCAT(node, _i) = -1; \
28 __typeof__(list->next) _##node = NULL; \
29 __typeof__(list->next) _next_##node = list->next; \
30 while (_next_##node) { \
32 __typeof__(list->next) node = _next_##node; \
33 _next_##node = (_next_##node)->next;
38#define LISTIFY(type) \
44#define ITEMIFY(type) \
48#define LIST_INIT(list) \
51 list->terminal = NULL; \
54#define LIST_ITEM_INIT(list) \
58#define LIST_APPEND(list, node) \
63 node->prev = list->terminal; \
64 if (list->terminal) { \
65 list->terminal->next = node; \
67 list->terminal = node; \
71#define LIST_PREPEND(list, node) \
75 list->terminal = node; \
77 node->next = list->next; \
78 list->next->prev = node; \
83#define LIST_REMOVE(list, node) \
84 if (list->terminal == node) { \
85 list->terminal = node->next \
89 if (list->next == node) { \
90 list->next = node->next; \
94 node->next->prev = node->prev; \
98 node->prev->next = node->next; \
102#define LIST_SORT(list, a, b, cmp_expr) { \
103 __typeof__(list->next) array[list->length]; \
104 LIST_FOREACH(list, node) \
105 array[node_i] = node; \
107 for (int i = 0; i < list->length; i++) { \
108 for (int j = i + 1; j < list->length; j++) { \
110 __typeof__(list->next) tmp = array[i]; \
111 array[i] = array[j]; \
116 if (list->length) { \
117 list->next = array[0]; \
118 list->terminal = array[list->length - 1]; \
122#define R(expr) PKRSRV_REF_BY(expr)